This is an introduction to programming using your Replit account and the p5.js library. p5.js allows you to use relatively simple JavaScript code to create interactive projects. The purpose of p5.js is to allow people to quickly and easily create art an interactive media projects on the web using JavaScript.
[1] Setup
In order to follow along you will need:
- A GitHub Account
- Be signed in to Replit.com using that GitHub Account.
We have covered this in previous classes so those steps are not in this tutorial but if you have questions or problems, let me know.
[2] Create a p5.js Repl
- Go to Replit.com. Make sure you are signed in using your GitHub Account.
- Click the Create button
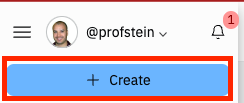
- Fill out the form and make sure you
- search for the p5.js template
- Choose p5.js template (NOT the one that also has Python in the name)
- Type in a name for your replit (this will also be your repository name)
- Click to create the Repl
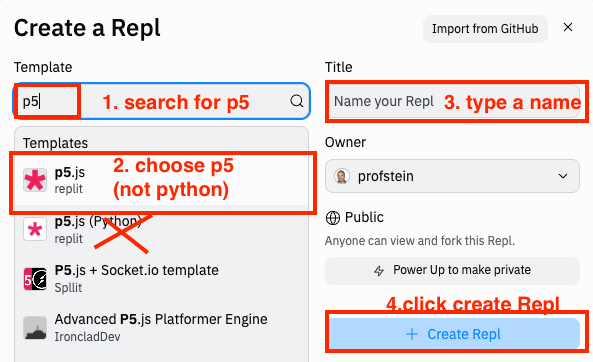
[3] Make Sure you have the Right Files and Everything is Working
You should have four files:
- script.js: this is where you will write your JavaScript
- index.html: this is the web page that will display what you do.
- README.md: this is the standard readme for the repl template. You can change this to fit your project.
- style.css: This is blank. You can use it to style your page if needed.
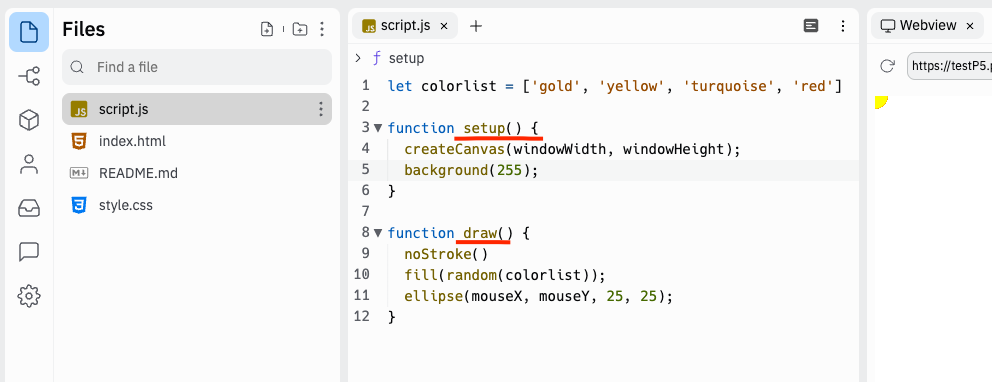
There is some default p5.js code that they add which should be active.
Move your mouse around the Webview screen and you should see it draw circles wherever you move your mouse.
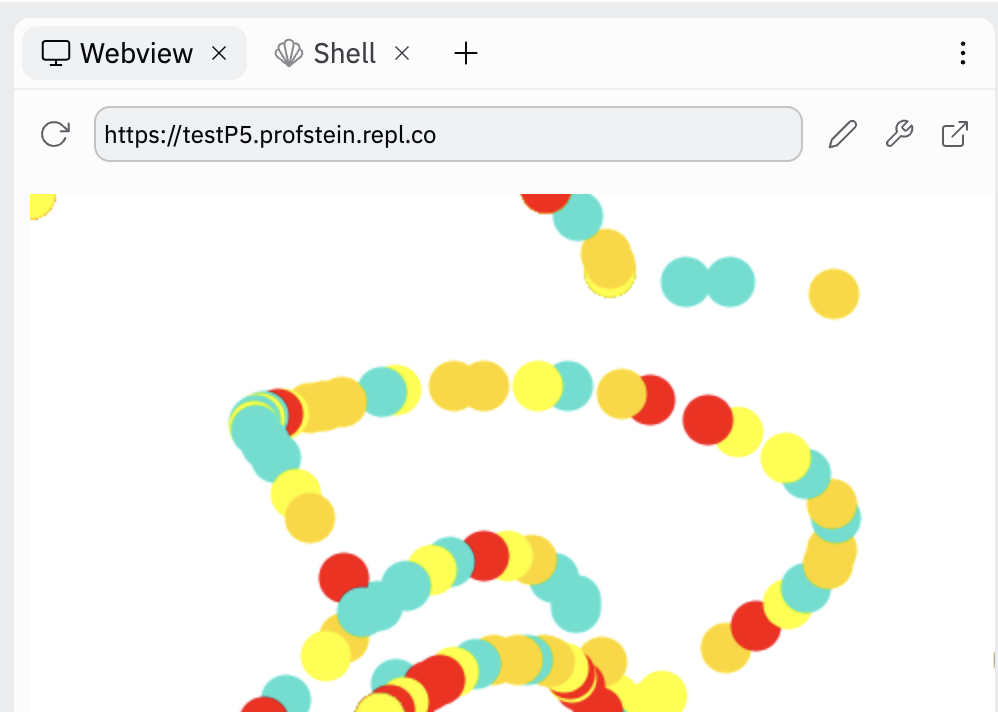
[4] Write Code and Test it Out
We will start in class by changing some colors.
At the top of the screen you can change which random colors are used by changing the colors in the colorlist array.
let colorlist = ['brown', 'green', 'orange', 'blue','pink','gold'];
Next try changing the background color by changing the number inside the parentheses on line 5.
background(155);
p5.js Reference
You can find out more info and copy code from the p5.js Reference.
[5] Code Examples You can Paste and Try
This code adds in an ellipse (circle) and lets you set the color (fill) and stroke color (stroke) as well as the thickness of the stroke (strokeWeight)
fill("#add8e6");
stroke("#ffff00");
strokeWeight(5);
ellipse(50,100,40,40);